Enhancing Code Quality with Pre-Commit Verification in NodeJS Applications
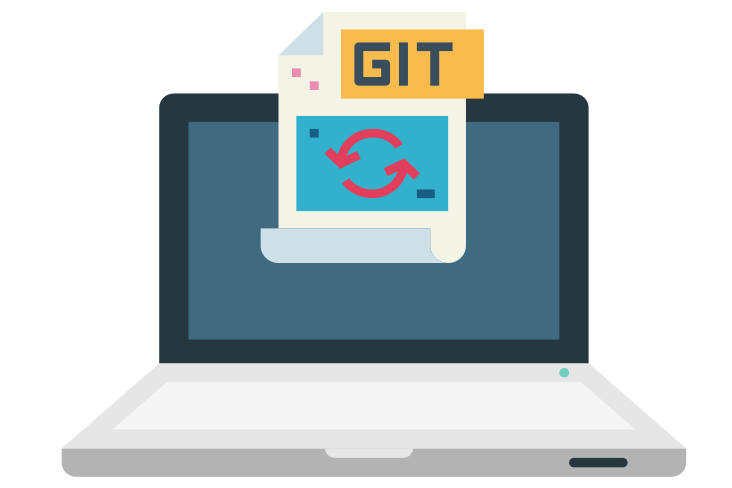
Maintaining high code quality is essential for any software project. One effective way to achieve this is by configuring your repository with pre-commit checks that automatically validate code before it’s committed. This saves a lot of time during code reviews and ensures that other engineers don’t have to worry about checking for adherence to standards.
Husky and lint-staged are tools that work in tandem to make this process seamless and efficient. In this article, we’ll explore how to set up a pre-commit check using Husky and lint-staged and understand the benefits it brings to the development workflow.
What are Husky and lint-staged?
Husky is a Git hook manager that allows developers to automate tasks before committing, pushing, or other Git actions. It enables you to define pre-commit hooks that run custom scripts, ensuring that certain conditions are met before a commit is allowed.
Lint-staged is a tool specifically designed to work with Husky. It focuses on optimising the linting process by running lint only on staged files, i.e., files that are part of the upcoming commit.
Setting up Husky and lint-staged
Step 1: Initialize the project and configure Husky
Make sure you have Node.js installed, then create a new project directory and initialize it with npm or yarn:
$ npx husky-init
$ npm install
Step 2: Install lint-staged
Next, install Husky and lint-staged as dev dependencies:
$ npm install --save-dev lint-staged
Inside .husky/pre-commit
add npx lint-staged
. Your file should look as follows:
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npx lint-staged
In your project’s .lintstagedrc.json
, add the following configuration:
{
"*.ts": [
"prettier --write",
"eslint"
],
"*.html": [
"eslint",
"prettier --write"
],
"*.scss": "prettier --write"
}
Step 3: Install commit lint
$ npm install --save-dev @commitlint/{config-conventional,cli}
configure the commit lint
echo "module.exports = {extends: ['@commitlint/config-conventional']}" > commitlint.config.js
add-hook
npx husky add .husky/commit-msg 'npx --no -- commitlint --edit ${1}'
Customization
You can customize the lint-staged section according to your project’s requirements. For example, you might want to include other lint like Prettier, stylelint, or TSLint for different file types.